Control your load prioritization in React
Let’s start talking about the idea behind bundle splitting in React , it is pretty simple as handling multiple bundles that can be dynamically loaded on demand at runtime. Basically, you should start:
- Importing all your dynamic imports as regular components with React.lazy
- Rendering all your lazy components inside a Suspense component.
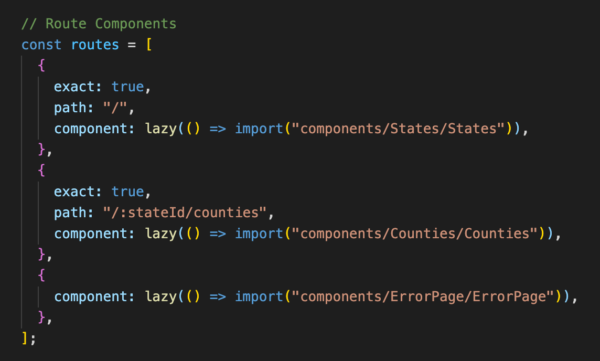
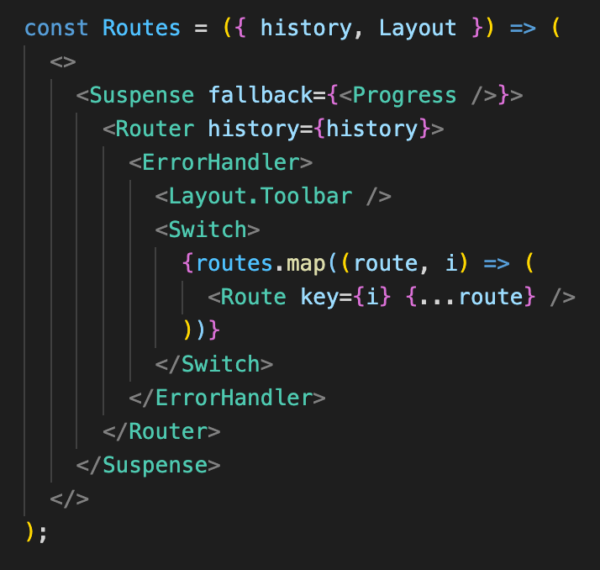
The outcome is pretty awesome too. You will end up with smaller bundles thanks to this control resource load prioritization approach, which generally give you a major impact on your React App load time.
Complete code
import React, { Suspense, lazy } from "react";
import PropTypes from "prop-types";
import { Router, Switch, Route } from "react-router-dom";
import { ErrorHandler } from "context/ErrorHandler";
import { Progress } from "components";
// Route Components
const routes = [
{
exact: true,
path: "/",
component: lazy(() => import("components/States/States")),
},
{
exact: true,
path: "/:stateId/counties",
component: lazy(() => import("components/Counties/Counties")),
},
{
component: lazy(() => import("components/ErrorPage/ErrorPage")),
},
];
const Routes = ({ history, Layout }) => (
<>
<Suspense fallback={<Progress />}>
<Router history={history}>
<ErrorHandler>
<Layout.Toolbar />
<Switch>
{routes.map((route, i) => (
<Route key={i} {...route} />
))}
</Switch>
</ErrorHandler>
</Router>
</Suspense>
</>
);
Routes.propTypes = {
history: PropTypes.object,
Layout: PropTypes.any,
};
export default Routes;